Level Up Your React Error Handling with Error Boundaries
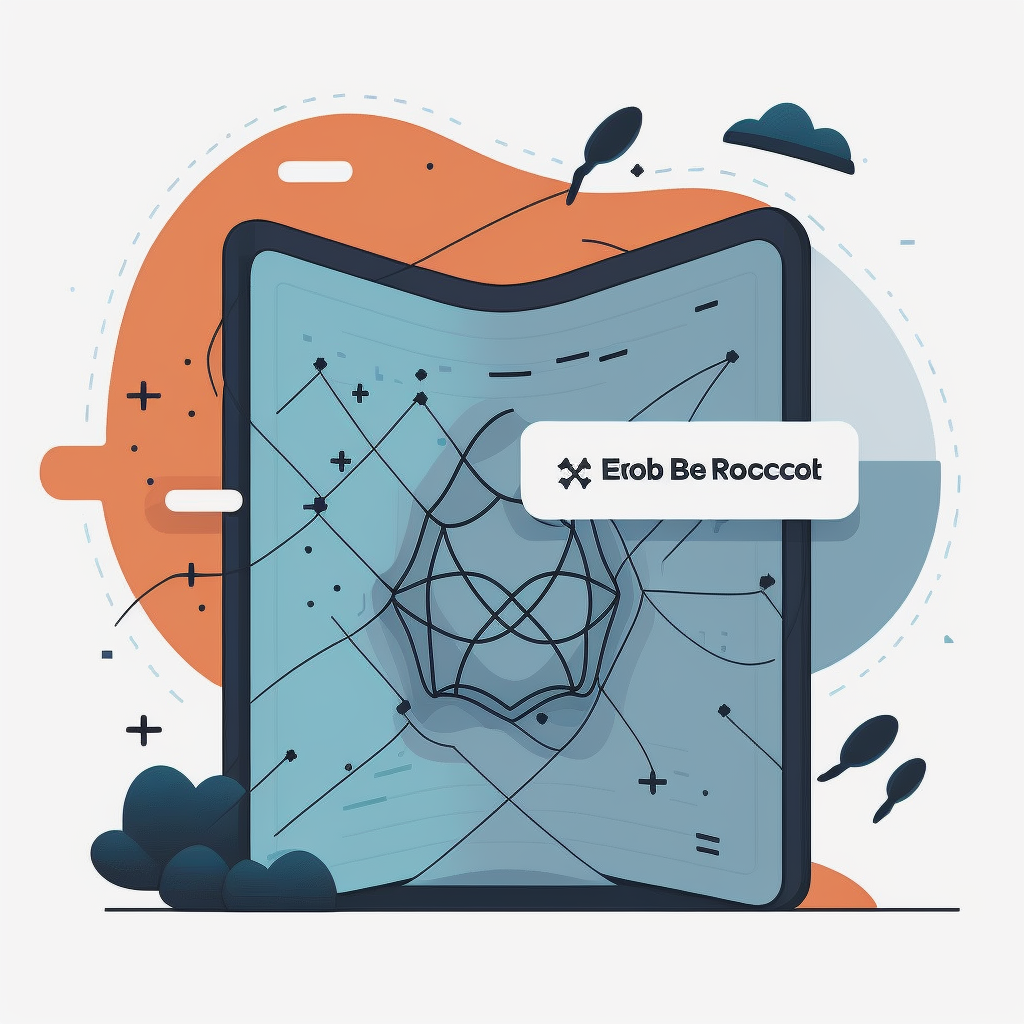
Hey there, fellow developer! π Error handling is one of those skills that can make a huge difference in your React applications, no matter where you are in your coding journey. We've all experienced that frustrating moment when our application crashes and displays a blank screen, leaving our users scratching their heads. But worry not, my friend! In this blog post, we will explore React's wonderful world of error boundaries and learn how they can help us create a more delightful user experience. Let's dive right in!
Understanding Error Boundaries
So, what exactly is an error boundary? Think of it as a superhero π¦ΈββοΈ that swoops in to save the day whenever there's trouble (i.e., a JavaScript error) in its child component tree. An error boundary catches those pesky errors, logs them, and displays a friendly fallback UI instead of the crashed component tree. It's like a safety net that keeps your app running smoothly, even when things go awry.
Creating an Error Boundary
Alright, it's time to create our very own error boundary. An error boundary is a class component that uses the built-in getDerivedStateFromError() method to update its state when an error occurs. Here's an example of a simple yet effective error boundary component:
class ErrorBoundary extends React.Component {
state = {
hasError: false
}
static getDerivedStateFromError() {
// Oh no, an error! Let's update our state.
return { hasError: true }
}
componentDidCatch(error, info) {
// We'll log the error and some useful info to the console for now.
console.error("Error:", error)
console.error("Info:", info)
}
render() {
// If there's an error, let's show the fallback UI.
// Otherwise, we'll render the child components as usual.
if (this.state.hasError) {
return this.props.fallback
}
return this.props.children
}
}
In this example, the getDerivedStateFromError() method is called when an error is thrown in any child components of the error boundary. When this happens, the method updates the state to inform us of an error. The componentDidCatch() method is used for logging and reporting the error, while the render() method displays the fallback UI if there has been an error or the child components if everything is A-OK.
Using Error Boundaries in Your React App
Now that we have our error boundary let's put it to work in our application! π οΈ You can wrap specific sections of your app in error boundaries to catch errors in those areas. For example, you might wrap each card in your UI with an error boundary to ensure that only the affected card displays a friendly error message rather than the entire application crashing and burning.
It's also a splendid idea to wrap your entire application in an error boundary. This ensures that users will always be notified if an error occurs anywhere in the app. Trust me, they'll appreciate the friendly error message much more than a blank screen!
Let's see some examples to see our error boundary in action! π
Wrapping individual components
Imagine a list of user profiles, each displayed in a ProfileCard component. We can wrap each ProfileCard with our ErrorBoundary to handle errors gracefully:
import ErrorBoundary from './ErrorBoundary'
function UserProfileList({ profiles }) {
return (
<div className="profile-list">
{profiles.map((profile) => (
<ErrorBoundary key={profile.id} fallback={<div>Oops, something went wrong with this profile.</div>}>
<ProfileCard profile={profile} />
</ErrorBoundary>
))}
</div>
)
}
If an error occurs in one of the ProfileCard components, only that specific card will display the error message, while the rest of the list remains unaffected.
Wrapping the entire application
We can also wrap our entire application in an ErrorBoundary to catch any unhandled errors:
import React from 'react'
import ReactDOM from 'react-dom'
import App from './App'
import ErrorBoundary from './ErrorBoundary'
ReactDOM.render(
<React.StrictMode>
<ErrorBoundary fallback={<h1>Oh no! Something went terribly wrong. Please try again later.</h1>}>
<App />
</ErrorBoundary>
</React.StrictMode>,
document.getElementById('root')
)
This way, if any error slips through the cracks, our users will still see a friendly error message instead of a blank screen.
Wrapping a component with async data fetching
Suppose we have a WeatherWidget component that fetches weather data asynchronously. We can wrap this component with our ErrorBoundary to display a fallback UI if the data fetching fails:
import React from 'react'
import WeatherWidget from './WeatherWidget'
import ErrorBoundary from './ErrorBoundary'
function WeatherDashboard() {
return (
<div className="weather-dashboard">
<ErrorBoundary fallback={<div>Sorry, we couldn't fetch the weather data. Please try again later.</div>}>
<WeatherWidget />
</ErrorBoundary>
</div>
)
}
If an error occurs during data fetching, our ErrorBoundary will catch it and display a helpful message to the user. These examples demonstrate the versatility and effectiveness of error boundaries in handling errors within your React applications. By using error boundaries strategically, you can create a more pleasant and resilient user experience. π